Autosender WhatsApp using Python
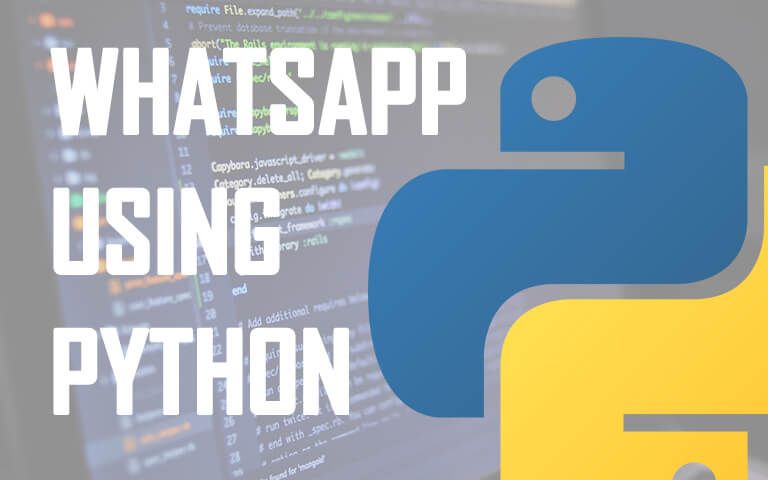
Probably you want to send automated message to a person or a group on Whatsapp, after browsing on the web, there are lots of working script on Python which allow us to send a message to a group.
As testing purposes, I have used a test script on Ubuntu 18.09 and on Raspberry PI (Rasbian 9.4) to “spam” a group of friends ^^ (For the RaspberryPI it was a bit longer..)
The main constraint is that the mobile phone with the WhatsApp app should to be on same network and connected as it uses the web interface of WhatsApp (https://web.whatsapp.com)
We need to authenticate after each connection on the browser by capturing the QR code on the mobile phone to authenticate the browser to use WhatsApp. (on my script i’m storing the cookies so it won’t ask me authentication on next login)
The script basically scan for html tags on the website using selerium and simulates the human behavior with codes executions.
As a requirement to execute the script: (Ubuntu 18.09 desktop)
- Python 2.7
- PIP module selenium (sudo pip install selenium)
- chromium-browser (sudo apt install chromium-browser)
- chromeDriver (download on http://chromedriver.chromium.org/downloads)
#!/usr/bin/python2
#Script to Auto Connect to Whatsapp WEB and send message to group or Individual
#Requirement selenium and chromium-browser which are available in PIP
#Driver chromedriver <http://chromedriver.chromium.org/downloads>
#Modules
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.common.by import By
import time
#Variables
#Replace with FULL path of chromedriver
#Driver chromedriver <http://chromedriver.chromium.org/downloads>
DRIVERPATH='/bin/chromedriver'
URL="https://web.whatsapp.com/"
WAITTIMEOUT=600
# Replace 'Friend's Name' with the name of your friend
# or the name of a group
target='"GroupName"'
# Replace the below string with your own message
string_message="TEST from Ubuntu"
#Message Loop
VALLOOP=1
SLEEPINTERVAL=3
#DIV for writing message in Whatsapp
#This Path Can change on WhatsAPP updates
inp_xpath = '//div[@class="_2S1VP copyable-text selectable-text"][@contenteditable="true"][@dir="ltr"][@data-tab="1"][@spellcheck="true"]'
x_arg = '//span[contains(@title,' + target + ')]'
#Save Session option for chrome - essentials for pages which requires authentication and avoid authentication on next login
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument("user-data-dir=selenium")
#workAround whatsapp on RPI
#chrome_options.add_argument("user-agent=Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/78.0.3904.70 Safari/537.36")
#headless browser
#chrome_options.add_argument("headless")
#Driver and options
driver = webdriver.Chrome(executable_path=DRIVERPATH, chrome_options=chrome_options)
#Connect to URL
driver.get(URL)
wait = WebDriverWait(driver, WAITTIMEOUT)
#Click on GROUP
group_title = wait.until(EC.presence_of_element_located((
By.XPATH, x_arg)))
group_title.click()
#Search for the input box to type message
input_box = wait.until(EC.presence_of_element_located((By.XPATH, inp_xpath)))
for i in range(VALLOOP):
input_box.send_keys(string_message + Keys.ENTER)
time.sleep(SLEEPINTERVAL)
#Close the Browser
driver.close()
The first login will open the a Browser and you will have to scan the QR on your mobile though WhatsAPP to authorise the browser. Afterwards, you can comment out the line
#chrome_options.add_argument(“headless”)
on next execution, the browser won’t open.
As a requirement to execute the script: (Raspberry PI – Rasbian 9.4 Stretch):
- Python 2.7
- PIP module selenium (sudo apt install python-pip -y && sudo pip install selenium)
- chromium-browser (sudo apt install chromium-browser)
- chromeDriver (sudo apt install chromium-driver)
The main ISSUE with chromium, the version that is found on the repo is Chromium v65 and WhatsApp no longer support this version (reference: https://groups.google.com/a/chromium.org/forum/#!topic/chromium-discuss/MbfH-OZGbLc)
As a working around, WhatsAPP only checks for User-Agent when connecting to its web, so I have forced the User-Agent to use another browser and it works
#!/usr/bin/python2
#Script to Auto Connect to Whatsapp WEB and send message to group or Individual
#Requirement selenium and chromium-browser which are available in PIP
#Driver chromedriver <http://chromedriver.chromium.org/downloads>
#Modules
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.common.by import By
import time
#Variables
#Replace with FULL path of chromedriver
#Driver chromedriver <http://chromedriver.chromium.org/downloads>
DRIVERPATH='/bin/chromedriver'
URL="https://web.whatsapp.com/"
WAITTIMEOUT=600
# Replace 'Friend's Name' with the name of your friend
# or the name of a group
target='"groupName"'
# Replace the below string with your own message
string_message="TEST from rpi"
#Message Loop
VALLOOP=1
SLEEPINTERVAL=3
#DIV for writing message in Whatsapp
#This Path Can change on WhatsAPP updates
inp_xpath = '//div[@class="_2S1VP copyable-text selectable-text"][@contenteditable="true"][@dir="ltr"][@data-tab="1"][@spellcheck="true"]'
x_arg = '//span[contains(@title,' + target + ')]'
#Save Session option for chrome - essentials for pages which requires authentication and avoid authentication on next login
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument("user-data-dir=selenium")
#workAround whatsapp on RPI
chrome_options.add_argument("user-agent=Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/78.0.3904.70 Safari/537.36")
#headless browser
#chrome_options.add_argument("headless")
#Driver and options
driver = webdriver.Chrome(executable_path=DRIVERPATH, chrome_options=chrome_options)
#Connect to URL
driver.get(URL)
wait = WebDriverWait(driver, WAITTIMEOUT)
#Click on GROUP
group_title = wait.until(EC.presence_of_element_located((
By.XPATH, x_arg)))
group_title.click()
#Search for the input box to type message
input_box = wait.until(EC.presence_of_element_located((By.XPATH, inp_xpath)))
for i in range(VALLOOP):
input_box.send_keys(string_message + Keys.ENTER)
time.sleep(SLEEPINTERVAL)
#Close the Browser
driver.close()
Predefine a custom User-Agent other than Chromium:
chrome_options.add_argument(“user-agent=Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/78.0.3904.70 Safari/537.36”)
As the Raspberry PI don’t have a desktop, we need to use X11 Forwarding for first authentication on whatsapp web (for me, it took some time to load the browser – don’t be in hurry ^^)
As a terminal, mobaxterm can be used
SSH -X <user>@server.com
#chrome_options.add_argument(“headless”)
Once the authentication is done, you can comment-out the line, to make automated script executions.
Some information about the scripts:
These DIV tag on the script are subjected to change on WhatsApp updates, so verify the web.whatsapp.com page source code to view the DIV that changed 😉
#DIV for writing message in Whatsapp
#This Path Can change on WhatsAPP updates
inp_xpath = ‘//div[@class=”_2S1VP copyable-text selectable-text”][@contenteditable=”true”][@dir=”ltr”][@data-tab=”1″][@spellcheck=”true”]’
x_arg = ‘//span[contains(@title,’ + target + ‘)]’
Happy Auto Whatsapping ^_^
Reference:
https://groups.google.com/a/chromium.org/forum/#!topic/chromium-discuss/MbfH-OZGbLc
http://chromedriver.chromium.org/downloads
https://www.geeksforgeeks.org/whatsapp-using-python/